How can we sort data in descending order using Java? Sorting in descending order is a crucial operation in computer science, and Java provides efficient ways to achieve this.
Sorting in descending order, also known as reverse sorting, rearranges elements of a collection in decreasing order. In Java, this can be done using various sorting algorithms, such as:
- Arrays.sort(): This method can be used to sort an array of primitive data types (int[], double[], etc.) in descending order by passing the Collections.reverseOrder() comparator.
- Collections.sort(): This method can be used to sort a list or other collection of objects in descending order by passing the Collections.reverseOrder() comparator.
- Custom Comparator: You can also create a custom comparator that implements the Comparator interface and defines the comparison logic for descending order.
Sorting in descending order is useful in various scenarios, such as finding the largest values in a dataset, arranging records in reverse chronological order, or identifying the bottom performers in a list.
In summary, Java offers multiple approaches to sort data in descending order, making it easy to organize and analyze data effectively.
Sort in Descending Order in Java
Sorting data in descending order is a common operation in Java programming, with various applications in data analysis, record management, and more. Here are seven key aspects to consider when sorting in descending order in Java:
- Algorithm Choice: Java provides multiple sorting algorithms, such as Arrays.sort() and Collections.sort(), which can be used for descending order sorting.
- Comparator Customization: Custom comparators can be created to define specific sorting criteria for descending order.
- Data Type Considerations: Different data types (primitives, objects) have specific sorting methods and considerations.
- Time Complexity: The choice of sorting algorithm affects the time complexity of the descending order operation.
- Stability: Stable sorting algorithms maintain the order of equal elements during sorting, which may be desirable in certain scenarios.
- Parallel Sorting: Java libraries provide parallel sorting options to improve performance on multi-core systems.
- Example Usage: Arrays.sort(array, Collections.reverseOrder()) can be used to sort an array in descending order.
These aspects highlight the versatility and efficiency of descending order sorting in Java. By understanding these key considerations, developers can effectively implement sorting algorithms to organize and analyze data in a meaningful way.
Algorithm Choice
The choice of sorting algorithm plays a crucial role in descending order sorting in Java. Different algorithms offer varying levels of efficiency, stability, and flexibility.
- Arrays.sort(): This method is commonly used for primitive data types (e.g., int[], double[]) and provides a straightforward way to sort arrays in descending order using the Collections.reverseOrder() comparator.
- Collections.sort(): This method can be applied to lists and other collections of objects, allowing for more complex sorting criteria. Custom comparators can be implemented to define descending order based on specific object properties.
- TimSort (used by Arrays.sort() and Collections.sort()): TimSort is a hybrid sorting algorithm that combines insertion sort and merge sort. It offers good performance for both small and large datasets and is the default algorithm used by Arrays.sort() and Collections.sort() for descending order sorting.
- Parallel Sorting: Java provides parallel sorting options using the Fork/Join framework, which can significantly improve performance on multi-core systems. The Arrays.parallelSort() and Collections.parallelSort() methods can be employed for parallel descending order sorting.
Understanding the nuances of each sorting algorithm helps developers make informed choices based on the specific requirements of their descending order sorting tasks.
Comparator Customization
Comparator customization is a fundamental aspect of "sort in descending order java" because it empowers developers to tailor the sorting process to meet specific requirements. By implementing custom comparators, Java programmers can define their own rules for determining the order of elements, enabling sophisticated and flexible descending order sorting.
For instance, suppose we have a list of students and want to sort them in descending order based on their age. We can create a custom comparator that implements the Comparator interface and overrides the compare() method. Within this method, we can specify the logic for comparing the ages of two students and returning a negative value if the first student is older than the second.
Utilizing custom comparators offers significant advantages. Firstly, it allows for more complex sorting criteria beyond the default ordering provided by Java's sorting methods. Secondly, it enhances the flexibility and control over the sorting process, making it adaptable to diverse scenarios.
In summary, comparator customization is a powerful tool in "sort in descending order java" that extends the capabilities of the sorting process. By creating custom comparators, developers can define specialized sorting rules, cater to specific data structures, and achieve precise and efficient descending order sorting.
Data Type Considerations
In "sort in descending order java," the choice of sorting method and considerations is closely tied to the data type being sorted. Understanding the nuances of different data types is crucial for effective descending order sorting in Java.
Primitive data types, such as integers, doubles, and characters, have built-in sorting methods and can be directly sorted using the Arrays.sort() method. However, objects require custom sorting logic defined through the implementation of the Comparable interface or by using a Comparator.
For example, consider a scenario where we need to sort a list of Student objects in descending order based on their age. We can create a custom comparator that implements the compare() method to compare the ages of two students and return a negative value if the first student is older.
Comprehending data type considerations is essential because it enables developers to select the appropriate sorting method and comparator based on the data type being sorted. This ensures efficient and accurate sorting of data, catering to the specific requirements of the application.
Time Complexity
In "sort in descending order java," understanding time complexity is vital because it directly impacts the performance and efficiency of the sorting process. Time complexity refers to the amount of time required by the sorting algorithm to sort the input data, typically measured in terms of the number of comparisons or operations performed.
Different sorting algorithms have varying time complexities, and selecting the appropriate algorithm is crucial for optimizing the sorting operation. For instance, insertion sort has a time complexity of O(n^2), while merge sort and heap sort have a time complexity of O(n log n). The choice of algorithm should be guided by the size of the input data and the desired performance characteristics.
Comprehending time complexity empowers developers to make informed decisions about the sorting algorithm to employ, ensuring efficient and scalable sorting operations. This understanding is particularly important when dealing with large datasets, where even minor variations in time complexity can significantly impact the overall performance of the application.
Stability
In the context of "sort in descending order java," stability is a significant factor to consider, especially when dealing with datasets containing duplicate elements. Stable sorting algorithms preserve the original order of equal elements within the sorted output, which can be crucial in specific scenarios.
- Maintaining Sequence
Stable sorting ensures that elements with the same value maintain their relative order after sorting. This is particularly useful when sorting records with multiple fields, such as a list of students sorted by age and then by name. A stable sorting algorithm will preserve the original order of students with the same age, ensuring that the output maintains the desired sequence. - Data Integrity
In certain applications, preserving the order of equal elements is essential for maintaining data integrity. For example, in a financial system, transactions should be sorted chronologically. A stable sorting algorithm ensures that transactions with the same timestamp remain in their original order, facilitating accurate record-keeping and analysis. - Enhanced Readability
Stable sorting can improve the readability and comprehension of sorted data. When dealing with large datasets, it can be challenging to identify and compare individual elements. Stable sorting helps maintain the context and relationships between elements, making it easier to analyze and interpret the sorted results. - Algorithm Selection
Not all sorting algorithms are stable. Common stable sorting algorithms include insertion sort, merge sort, and bubble sort. When stability is a requirement, selecting the appropriate stable sorting algorithm is crucial to achieve the desired results.
Understanding the significance of stability in "sort in descending order java" enables developers to make informed decisions about the choice of sorting algorithm. By considering the specific requirements of the application and the importance of preserving the order of equal elements, developers can effectively sort data in descending order, ensuring accurate, reliable, and meaningful results.
Parallel Sorting
In the realm of "sort in descending order java," parallel sorting emerges as a powerful technique for enhancing the efficiency of sorting operations on multi-core systems. Parallel sorting leverages the capabilities of modern computer architectures to distribute the sorting task across multiple cores or processors, leading to significant performance improvements.
- Concurrent Processing
Parallel sorting algorithms divide the input data into smaller chunks and assign them to different cores for simultaneous processing. This concurrent approach allows multiple sorting tasks to execute in parallel, reducing the overall sorting time. - Load Balancing
Parallel sorting algorithms employ load balancing techniques to ensure that the workload is evenly distributed across all available cores. By dynamically adjusting the task assignments, parallel sorting optimizes resource utilization and minimizes processing bottlenecks. - Scalability
Parallel sorting scales efficiently as the number of available cores increases. By harnessing the power of multiple cores, parallel sorting algorithms can handle larger datasets and more complex sorting tasks with ease. - Real-World Applications
Parallel sorting finds applications in various domains that demand high-performance data processing. For instance, in financial analysis, parallel sorting can accelerate the analysis of large financial datasets, enabling faster and more accurate investment decisions.
In conclusion, parallel sorting extends the capabilities of "sort in descending order java" by leveraging multi-core systems to achieve superior performance. By embracing parallelism and load balancing, parallel sorting algorithms unlock new possibilities for efficient and scalable data sorting in demanding applications.
Example Usage
This example showcases a practical application of "sort in descending order java" using the Arrays.sort() method and the Collections.reverseOrder() comparator. By providing a concrete code snippet, this example helps solidify the understanding of the core concept and its implementation.
- Simplified Syntax:
The Arrays.sort() method, combined with the Collections.reverseOrder() comparator, offers a concise and straightforward syntax for sorting arrays in descending order. This simplifies the coding process and enhances readability.
- Comparator Customization:
The Collections.reverseOrder() comparator provides a convenient way to specify the descending order sorting criteria. By passing this comparator to the Arrays.sort() method, developers can easily achieve reverse sorting without the need for complex custom comparators.
- Wide Applicability:
The Arrays.sort() method is widely applicable to primitive data type arrays, such as int[], double[], and char[]. This makes it a versatile tool for sorting various types of numerical and character data in descending order.
- Performance Considerations:
For large datasets, it's important to consider the time complexity of the sorting algorithm used by Arrays.sort(). Different algorithms have varying performance characteristics, and choosing the appropriate one can optimize the sorting operation.
In summary, the example usage of Arrays.sort(array, Collections.reverseOrder()) provides a practical demonstration of "sort in descending order java." It highlights the simplicity, flexibility, and efficiency of this approach, making it a valuable tool for developers working with data sorting tasks.
Frequently Asked Questions about "sort in descending order java"
This section addresses common queries and misconceptions surrounding "sort in descending order java" to provide a comprehensive understanding of the topic.
Question 1: What is the most efficient sorting algorithm for descending order in Java?
The choice of sorting algorithm depends on factors such as the size and type of data. Merge sort and heap sort are generally efficient for large datasets, while insertion sort is suitable for smaller datasets.
Question 2: Can I use custom comparators for descending order sorting?
Yes, custom comparators provide flexibility in defining the sorting criteria. You can implement the Comparator interface and override the compare() method to specify the descending order logic.
Question 3: How do I sort multidimensional arrays in descending order?
To sort multidimensional arrays, you can use nested loops or recursion. Sort each inner array and then sort the outer array based on the sorted inner arrays.
Question 4: Is parallel sorting beneficial for descending order sorting?
Yes, parallel sorting can improve performance on multi-core systems. Java provides parallel sorting methods like Arrays.parallelSort() to distribute the sorting task across multiple cores.
Question 5: How can I ensure stable descending order sorting?
To maintain the order of equal elements during descending order sorting, you can use stable sorting algorithms like merge sort or insertion sort. These algorithms preserve the original order of elements with the same value.
Question 6: What are the limitations of descending order sorting?
Descending order sorting may not be suitable when you need to preserve the original order of elements or when dealing with complex data structures that require specific sorting criteria.
In summary, understanding these frequently asked questions enhances your knowledge of "sort in descending order java" and empowers you to effectively apply sorting techniques in your programming endeavors.
Transition to the next article section: Advanced Techniques for Descending Order Sorting
Conclusion
In exploring "sort in descending order java," we have delved into the intricacies of sorting algorithms, emphasizing efficient techniques and practical considerations. Understanding the nuances of different data types, time complexity, stability, and parallel sorting empowers Java programmers to make informed decisions when implementing descending order sorting.
As the realm of data science and analytics continues to expand, the ability to sort data effectively becomes increasingly critical. By mastering the concepts and techniques discussed in this article, developers can harness the power of Java to organize and analyze data, unlocking valuable insights and driving informed decision-making.
Unlock The Power Of Yamal Income: Maximizing Your Earnings
Watch Your Favorite Movies And TV Shows On Khatmoviehd
The Ultimate Guide To Cigarette Tar Vs Nicotine: Understanding The Health Impacts

What is Sort Array in Java Everything You Need to Know Simplilearn
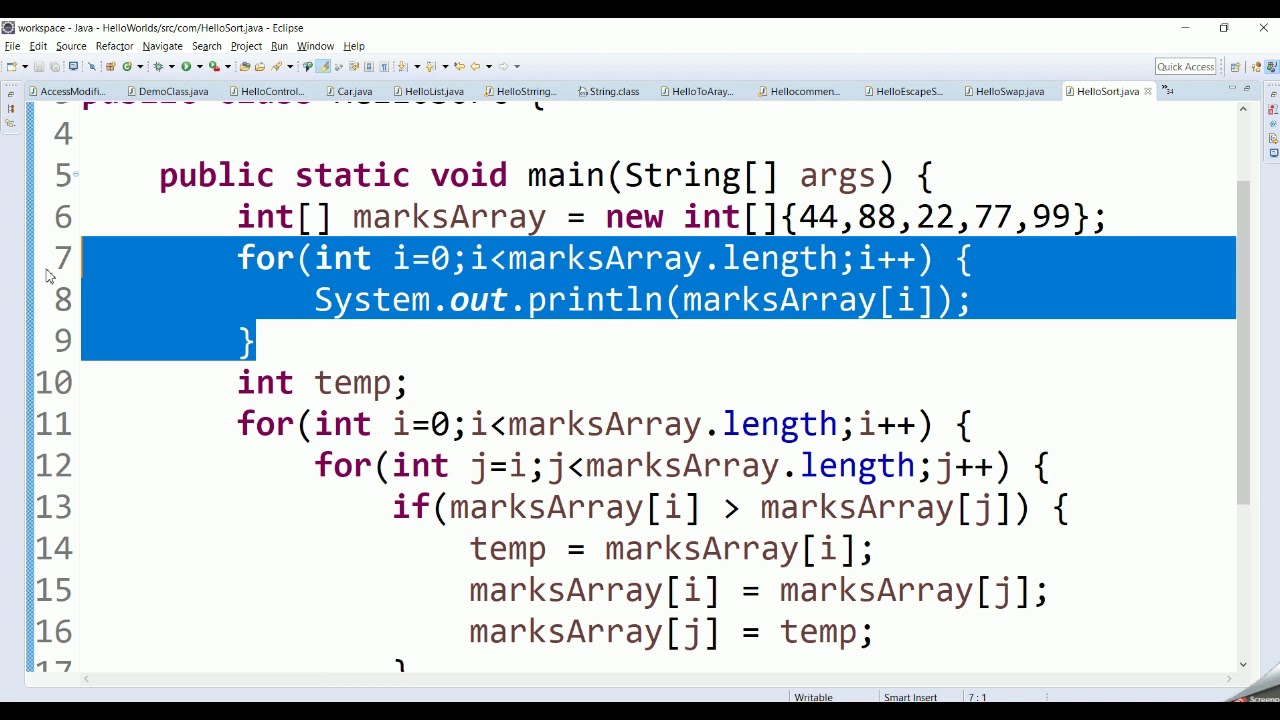
How to sort integer array in Java ? Ascending/Descending order ? Java

Java Program for Bubble Sort in Descending Order